Technical Blog
JS Fundamentals
Learning Competencies
Control flow and Loops, Arrays and Objects
Control flow
The control flow is the order in which the computer executes statements in a script. Code is run in order from the first line in the file to the last line, unless the computer runs across the (extremely frequent) structures that change the control flow, such as conditionals and loops.
Control flow statements are those that make decisions about what happens in a program. A programmer needs to take care that the conditions used do not contradict one another. This would create regions in the code that would be unreachable.
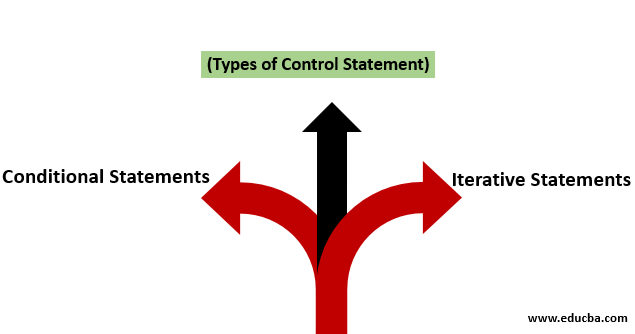
- Conditional Statements: based on an expression passed, a conditional statement makes a decision, which results in either TRUE or FALSE.
- Iterative Statements (Loop): Until and unless the expression or the condition given is satisfied, these statements repeat themselves.
if (condition)
{
// code to be executed of condition is true
}
else {
// code to be executed of condition is false
}
Loops
Have you ever been punished in school and asked to write the sentence “I will not be late for the class again.” fifty times? It’s so boring, right? Well, JavaScript saves us from this trouble. When we ask JavaScript to execute the same task repeatedly, it’s a lot more efficient than us. How so? Simple, JavaScript uses loops! This tutorial is all about what JavaScript loops are and how we can use them. In this article, we will learn about different types of loops available in JavaScript. These include for, while and do…while loops. There are also some loops that are special in JavaScript, like for…in and for…of loops. Let’s learn about all the loops with their syntax and examples.
What is a JavaScript Loop?
JavaScript Loop provides a quick and easy way to do repetitive tasks. They offer to perform iterations with only a few lines of code. Iteration is the number of times you want to repeat the task (that number can even be zero). Be sure you understand all of them, so you can implement the best loop statement for a given situation.
There are primarily two types of loops in any programming language, and JavaScript is no exception. These are:
- Entry Controlled Loops: Any loop where we check the test condition before entering the loop is an entry controlled loop. In these loops, the test condition determines whether the program will enter the loop or not. These include for, while, etc.
- Exit Controlled Loops: Any loop where we check the test condition after the statements are executed once is an exit controlled loop. In these loops, the test condition determines whether or not the program will exit the loop. This category includes do…while loop.
We repeat the sequence of instructions until the test condition is true. JavaScript provides the following loop statements to achieve this:
- while statement
- for statement
- do..while statement
- for…in statement
- for…of statement
- labeled statement
- break statement
- continue statement
while Statement
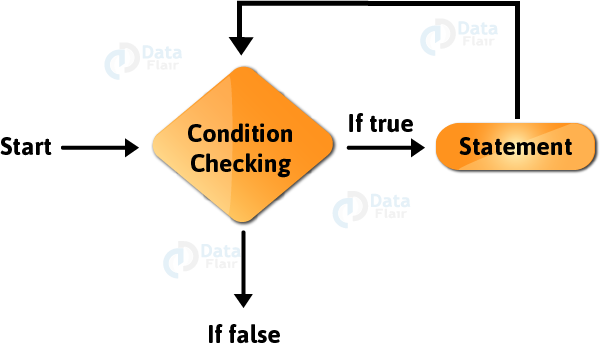
A while statement in JavaScript executes until our boolean condition evaluates to true. This loop is an entry controlled loop.
for Statement
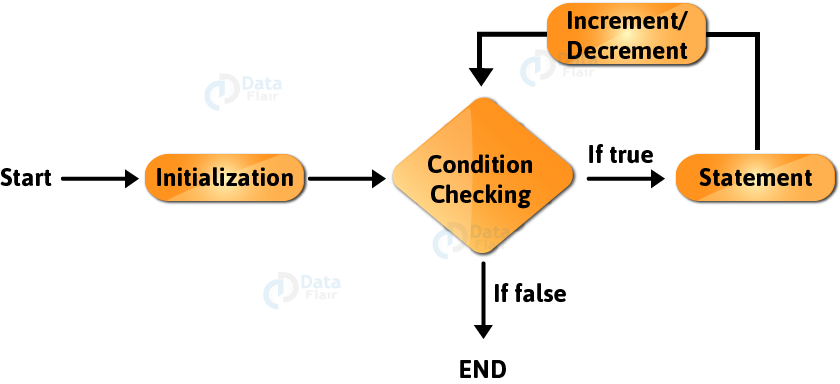
JavaScript for loops are similar to Java and C language for loops. It’s an entry controlled loop. It consists of three parts, separated with a semicolon:
- Initialization: It initializes the loop with an iteration variable and executes once at the beginning of the loop.
- Condition: It specifies a certain condition in the loop that determines whether the loop body will execute or not. If the condition returns true, the code inside the for loop will execute. If it returns false, the control moves onto the statement after the loop.
- Increment/ Decrement: This section increments or decrements the value of our iteration variable by 1.
do..while Statement
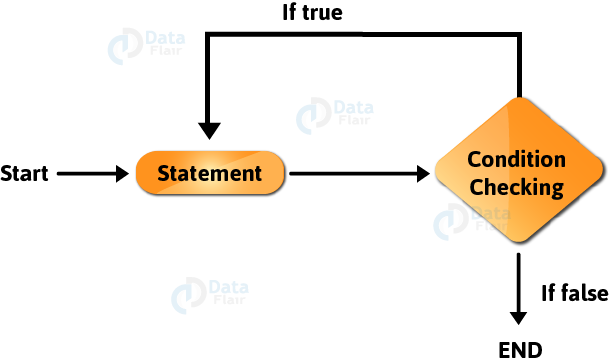
This is an exit controlled loop; the loop body executes at least once, even if the condition is not true. This is because the condition is tested when the loop body ends. If the condition returns true, the code inside the loop executes again. If it returns false, the JavaScript interpreter exits the loop.
for…in Statement
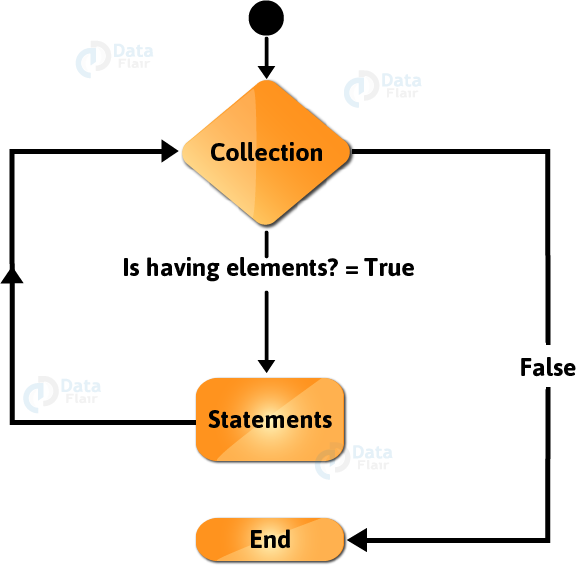
This is a modified for loop that doesn’t require the programmer to know the number of iterations the loop needs to perform. It creates a loop iterating over all the enumerable properties of an object. The keys store the key of the current property and we access the property’s value with it. This means that the loop executes a specific set of statements for each distinct property in a JavaScript Object. The working of a for…in statement in JavaScript is similar to for-each loop in Java.
The reason why the developers created the for…in loop is to work with user-defined properties in an object. It is better to use a traditional for loop over Array elements with numeric indexes.
for…of Statement
In the last JavaScript statement, we had to access the array’s values with the help of square brackets [ ]. We couldn’t access them directly and it makes your program more error-prone. So the JavaScript developers got us a new way to access these values directly. This makes our code a lot more efficient than before because the values store the property’s value, not the key.
Difference between for and while loop in JavaScript
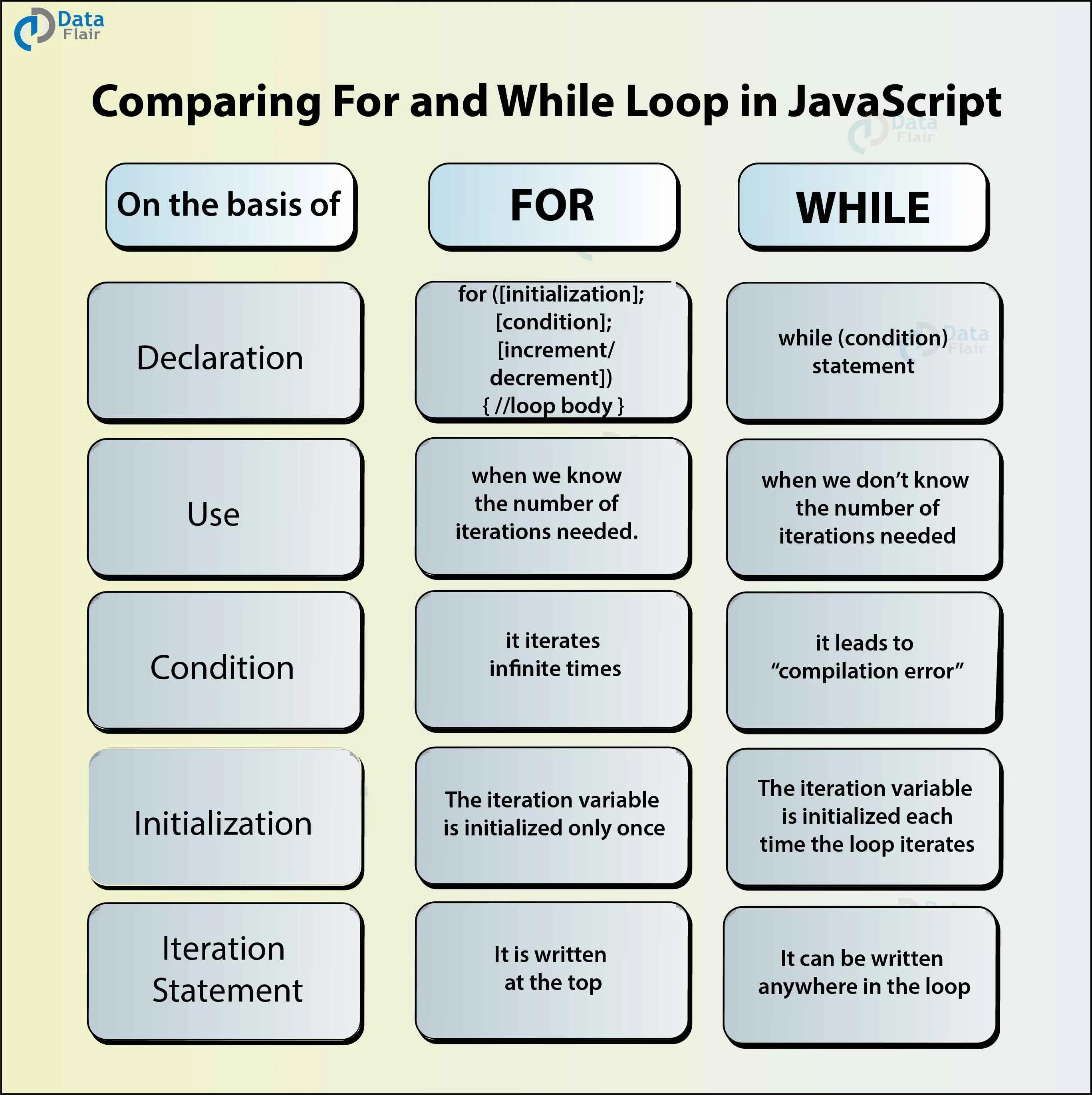
What are JavaScript Objects?
Objects are the basic building blocks for every object-oriented language. JavaScript being an object-oriented language, is no exception to this concept. With javascript being widely adopted due to its ability to provide dynamic behaviour to web pages, one should be aware of javascript and how to work with its objects. Objects in javascript are a group of different data types or objects put together as “key-value” pairs. The “key” part of the object is nothing but the object properties.
How to Create an Object in JavaScript?
Example
const person = {
firstName: "John",
lastName: "Doe",
age: 50,
eyeColor: "blue"
};
JavaScript Object Methods
create(): As we have already seen above, this method is used to create javascript objects from a prototype object.
is(): This method takes in a second object as a parameter and determines if both the objects are equal and return a Boolean value. That is, if both objects are equal, then “true” is returned, else “false”.
keys(): The keys() method takes in the javascript object as a parameter and returns an array of its properties.
values(): Similarly, the values method takes in a javascript object as a parameter and returns an array of its values.
entries(): This method also takes in a javascript object as a parameter and returns an array containing another array of both key-value pairs.
What is JavaScript Array?
The definition of an array in Java goes something like this: “An array is a collection of homogeneous elements, stored in a contiguous memory location.”
In the last JavaScript statement, we had to access the array’s values with the help of square brackets [ ]. We couldn’t access them directly and it makes your program more error-prone. So the JavaScript developers got us a new way to access these values directly. This makes our code a lot more efficient than before because the values store the property’s value, not the key.
The array can be created in JavaScript, as given below
var country = [ "India" , "England" , "Srilanka" ];
In the above creation, you are initializing an array, and it has been created with values “India”, “England”, and “Srilanka”. The index of “India”, “England”, and “Srilanka” is 0, 1, and 2, respectively.
The Difference Between Arrays and Objects
In JavaScript, Arrays use numbered indexes.
In JavaScript, Objects use named indexes.
When to Use Arrays. When to use Objects.
JavaScript does not support associative Arrays.
You should use Objects when you want the element names to be strings (text).
You should use Arrays when you want the element names to be numbers.